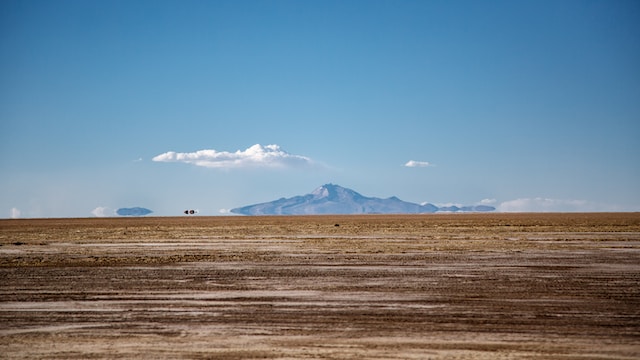
API testing and frontend development often rely on mock APIs to simulate backend behavior. MirageJS is an excellent tool that simplifies the creation of realistic mock APIs for JavaScript applications. In this blog post, we will explore the features and benefits of MirageJS through a practical example. By the end, you’ll have a clear understanding of how MirageJS can enhance your development workflow.
What is MirageJS?
MirageJS is a client-side API mocking library that intercepts requests made by the frontend and provides customizable responses. It allows developers to build mock APIs that closely mimic real APIs, complete with routes, data models, and response customization.
Setting up MirageJS:
To get started with MirageJS, follow these simple steps:
1. Install MirageJS:
|
|
2. Configure MirageJS
In your application’s entry point (e.g., index.js
or main.js
), import MirageJS and define your mock API endpoints and responses.
|
|
In this example, we defined a GET route for /api/posts
that returns an array of blog posts. Additionally, we defined a POST route for /api/posts
that accepts a new post object in the request body and returns the newly created post with a unique ID assigned.
Using the Mock API in Your Application:
With MirageJS set up and routes defined, you can now use the mock API in your application code.
|
|
In this example, we use the fetch
API to retrieve the mock blog posts from /api/posts
. The response is logged to the console, demonstrating how MirageJS intercepts the request and provides the defined mock data.
MirageJS is a powerful tool that simplifies the creation of realistic mock APIs for API testing and frontend development. By configuring routes and responses, you can simulate various scenarios and focus on building and testing your frontend without relying on a backend API. With MirageJS, you have full control over your mock APIs, enabling faster development cycles and more reliable testing. Give MirageJS a try in your next project and experience the benefits firsthand. Happy mocking!